I started working on my first Objective-C project this weekend. I spent most of the week watching videos on Lynda.com by Simon Allardice covering the basics of iPhone development. The goal is to eventually build VoidTrader on the iPhone/iPad. For now though, I'm going to build a small RPG fighting game. I have a few iterations planned, which I may put up in a different post. Below is an example of the Dice class I created. This initial iteration served a couple of goals. First, I built a main screen, about screen, and combat screen. This allowed me to get comfortable with adding different views. Next the Dice class itself allowed me to practice building and using a custom class.
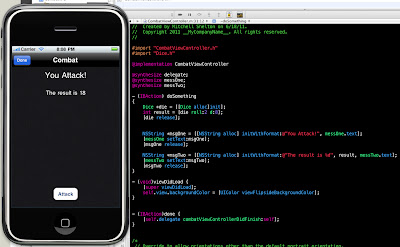
Obviously, I intend on adding a lot more to that screen. I would like to do some custom graphics and perhaps animations. However, that will have to wait for several iterations.
The Dice class:
// // Dice.m // CombatRPG // // Created by Mitchell Shelton on 6/18/11. // Copyright 2011 MitchellShelton.com. All rights reserved. // #import "Dice.h" @implementation Dice /* * A Dice simulator method * @numDie * The number of dice being rolled * @sides * The number of sides on the dice */ - (int)roll :(int)numDie d:(int)sides { // Handle incorrect dice or sides if (numDie == 0) { // Is the quantity zero? // There must be at least one die numDie = 1; } if (sides == 0 || sides == 1) { // Are the sides zero or one? // Any die must have at least two sides sides = 2; } // Initialize our return value int result = 0; // Loop over the die rolls for (int i=0; i < numDie; i++) { // Roll the die int rollResult = (arc4random() % sides) + 1; // Add the die roll to our total result result = result + rollResult; } // Return the result of our roll return result; } @end
Calling the method:
Dice *die = [[Dice alloc]init]; int result = [die roll:2 d:8]; [die release];
So, why a dice class?
I'm basing the RPG "engine" on some modified Dungeons and Dragons rules for combat, encounters, and general mechanics. So there will be initiative rolls, weapons will do damage based on rolls, etc. I already wrote classes for the dice, players, armor, and weapons in PHP. I also have all of the stats for experience points, a few default weapons types, and miscellaneous other data that will be kept in a database.
So what is next?
I'm going to get that data from a remote MySQL database into my iPhone app. This will come via a web service eventually, but for now it will most likely come from flat xml files. That will allow me to focus on the iPhone end of programming, and not on figuring out the web service part. Eventually, I will need the web service to be writable though so I can allow users to upload profiles to a connected web site.
-Mitchell